Dataset 가져온 출처: https://www.kaggle.com/c/titanic
Titanic - Machine Learning from Disaster | Kaggle
www.kaggle.com
Machine Learning 분석을 적용하여 어떤 사람들이 타이타닉호에서 생존할 수 있었는가에 대해 분석해봄.
ㅇ 활용 모델
- Logistic Regression
- k-Nearest Neighbors
- Support Vector Machines
- Naive Bayes classifier
- Decision Tree
- Artificial neural network
1. 데이터 전처리
kaggle에서 다운받은 excel의 데이터가 공백, 데이터형식 등 다른 부분이 많다.
그것들을 정제하기 위한 절차를 수행
1-1. 함수 선언 및 데이터 확인
1) 함수 선언
# 데이터 분석
import pandas as pd
import numpy as np
import random as rnd
# 시각화
import seaborn as sns
import matplotlib.pyplot as plt
%matplotlib inline #주피터 노트북 상 그래프 표현위한 라인
# 기계 학습
from sklearn.linear_model import LogisticRegression
from sklearn.svm import SVC, LinearSVC
from sklearn.ensemble import RandomForestClassifier
from sklearn.neighbors import KNeighborsClassifier
from sklearn.naive_bayes import GaussianNB
from sklearn.linear_model import Perceptron
from sklearn.linear_model import SGDClassifier
from sklearn.tree import DecisionTreeClassifier
from sklearn.neural_network import MLPClassifier
2) 데이터 로딩 및 확인
# 데이터 로딩 // df: dataframe 데이터 읽음
train_df = pd.read_csv('./train.csv')
test_df = pd.read_csv('./test.csv')
combine = [train_df, test_df] // 데이터 합침
print(train_df.columns.values) // values 열 출력
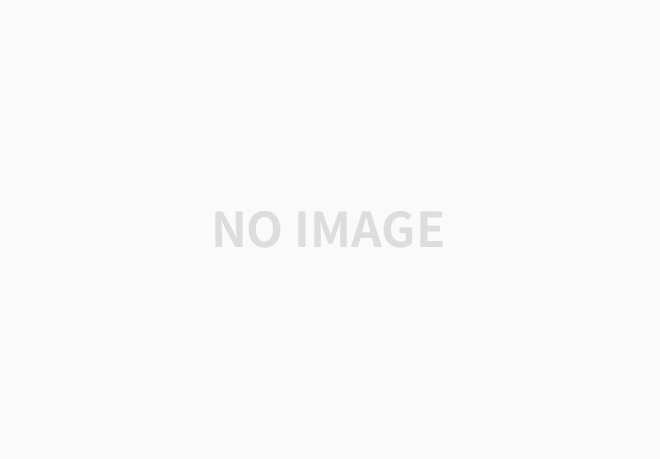
# preview the data
train_df.head() // method를 불러서 예쁘게 보여줌. head(): 5lines까지만 보여줌.
train_df.tail() // tail(): 뒤에서 5lines 보여줌.
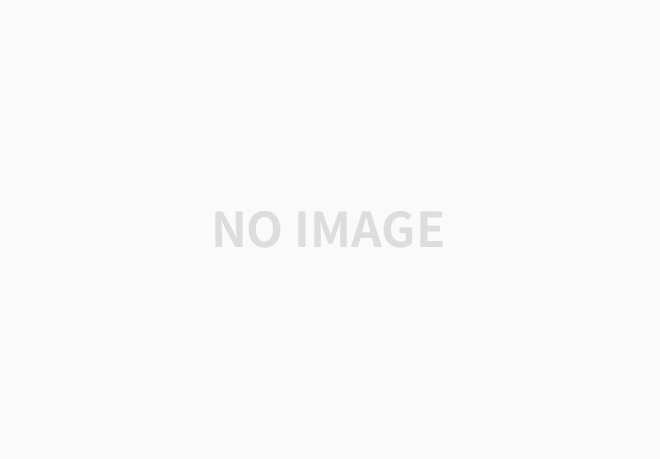
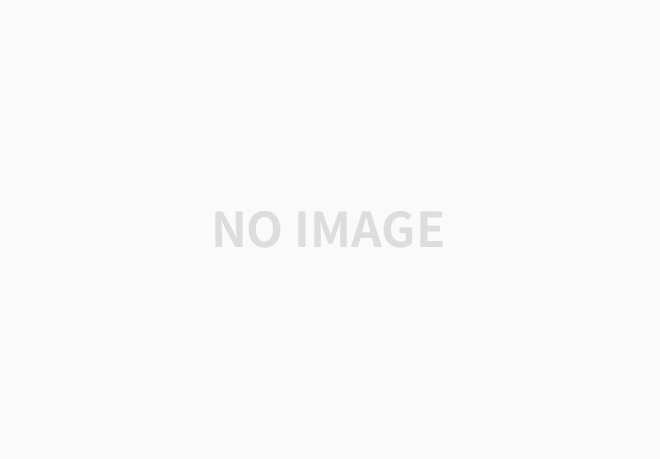
# train data와 test data info 출력
train_df.info()
print('_'*40)
test_df.info()
train_df.describe()
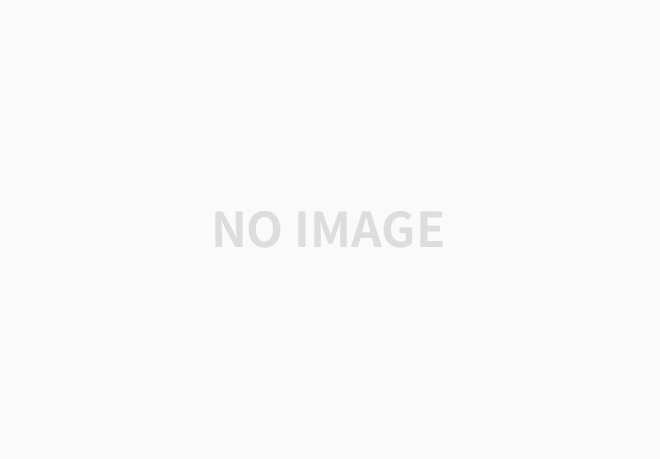
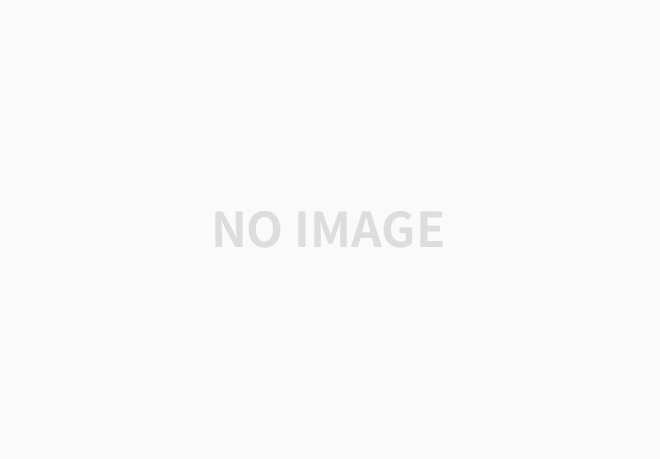
1-2. Missing Value 처리 - Data 삽입을 위해 기존 Data의 중간값 / 평균값 구하기
# check missing values in train, test dataset
train_df.isnull().sum()
test_df.isnull().sum()
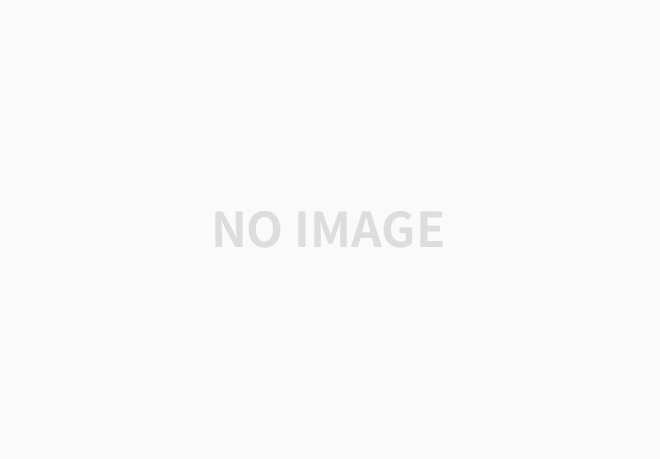
missing된 value는 age, cabin, 그리고 test dataset의 Fare.
# training data의 age, cabin의 경우 누락 값들은 전체 값 중 몇프로일까?
sum(pd.isnull(train_df['Age']))/len(train_df["PassengerId"]) // 전체 length에서 null값의 갯수를 나눈다.
sum(pd.isnull(train_df['Cabin']))/len(train_df["PassengerId"])
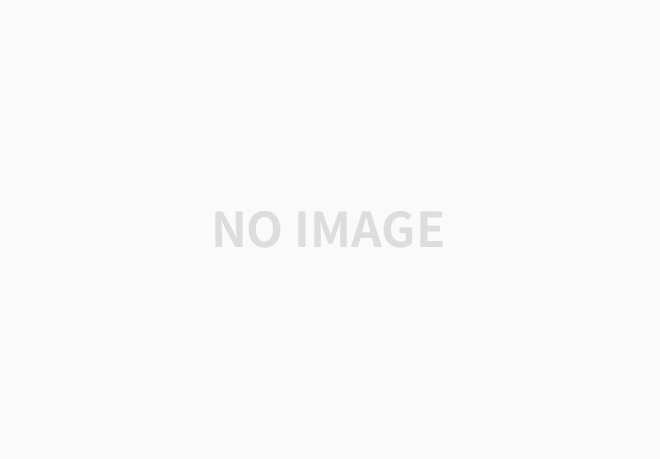
# Train의 Age분포를 히스토그램으로 확인해보자
ax = train_df["Age"].hist(bins=15, color='teal', alpha=0.8) // bins=bar 갯수, alpha=투명도
ax.set(xlabel='Age', ylabel='Count') // x, y축 레이블
plt.show()
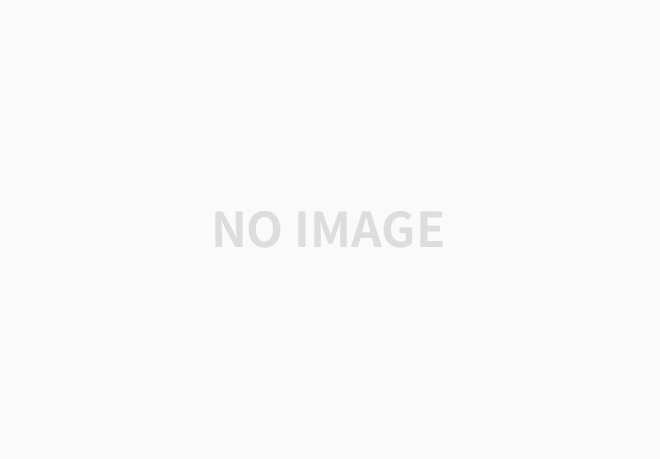
# 중간값은 약 20-30대로 보인다. 정확한 중간값을 알아보자
train_df["Age"].median(skipna=True)
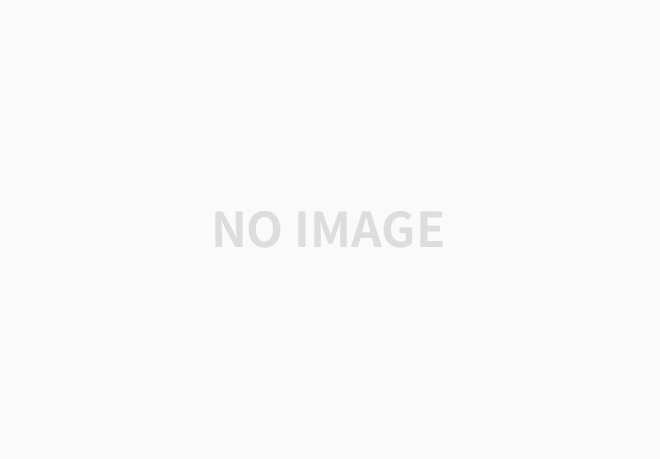
글쿤.
# 이번엔 train data의 Embarked 중간값을 알아보자
countplot 하나 써보장. 딱봐도 S가 제일 많다
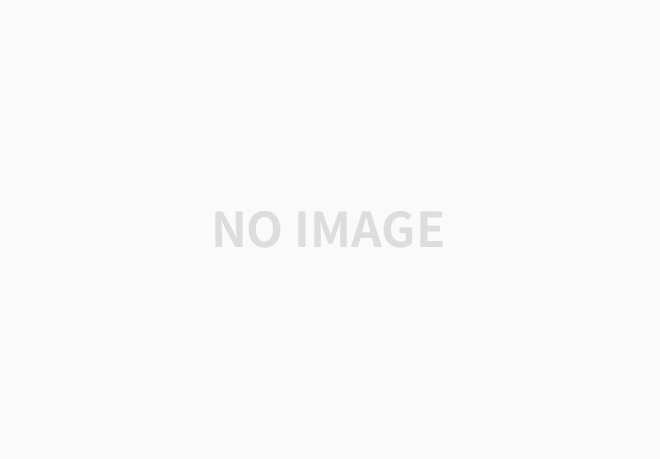
# 마지막으로 Fare의 평균값은?
train_df["Fare"].mean(skipna=True)
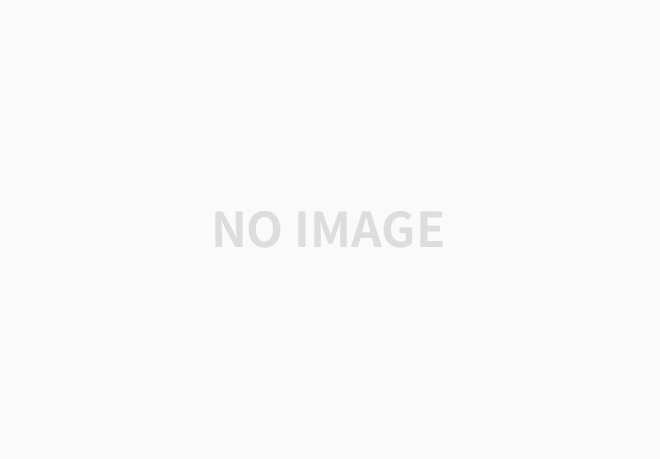
정수써야하므로 32로 생각하자.
1-3. Missing Value 처리 - Data입력
- Embarked는 S가 가장 많으니 누락값에 S를 채워넣고,
- Fare는 평균값인 32를 취해서 누락값에 넣는다. (항구/티켓에 따라 다르지만 편의상)
- Cabin은 누락값 많았지만, 값들이 char형으로 평균/중간값 구하기 애매함.
그래서 Cabin 속성 제거.
# 누락된 값을 적절한 값으로 채워넣기
train_df["Age"].fillna(28, inplace=True)
test_df["Age"].fillna(28, inplace=True)
train_df["Embarked"].fillna("S", inplace=True)
test_df["Fare"].fillna(32, inplace=True)
# 누락된 값이 너무 많은 속성 제거
train_df.drop('Cabin', axis=1, inplace=True)
test_df.drop('Cabin', axis=1, inplace=True)
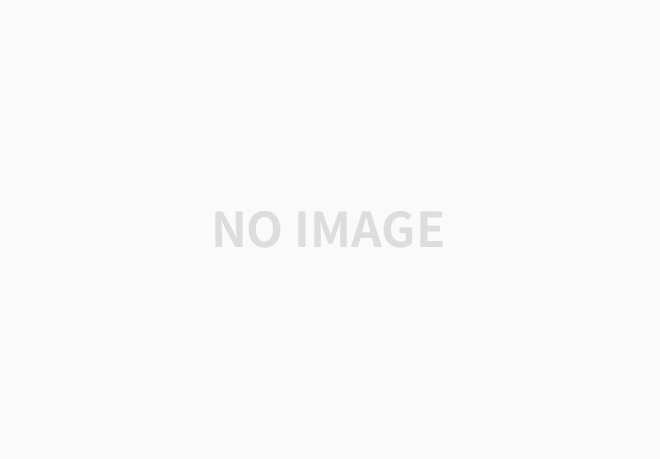
1-4. 데이터 속성별 값에 따라 생존자 확인
# 각 속성(열)에 따른 생존자 확률
train_df[['Pclass', 'Survived']].groupby(['Pclass'], as_index=False).mean().sort_values(by='Survived', ascending=False)
train_df[["Sex", "Survived"]].groupby(['Sex'], as_index=False).mean().sort_values(by='Survived', ascending=False)
train_df[["SibSp", "Survived"]].groupby(['SibSp'], as_index=False).mean().sort_values(by='Survived', ascending=False)
train_df[["Parch", "Survived"]].groupby(['Parch'], as_index=False).mean().sort_values(by='Survived', ascending=False)
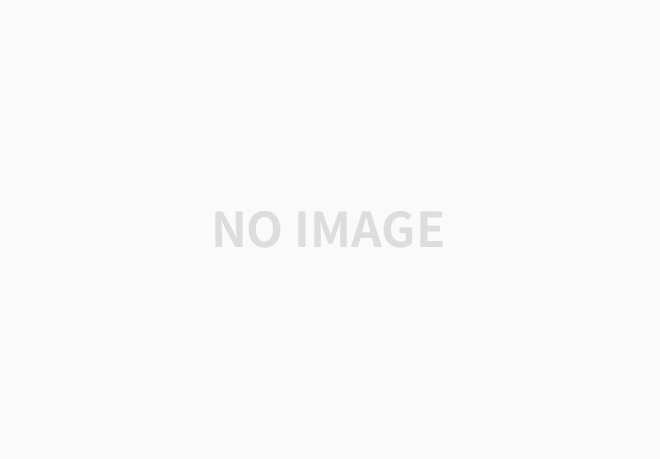
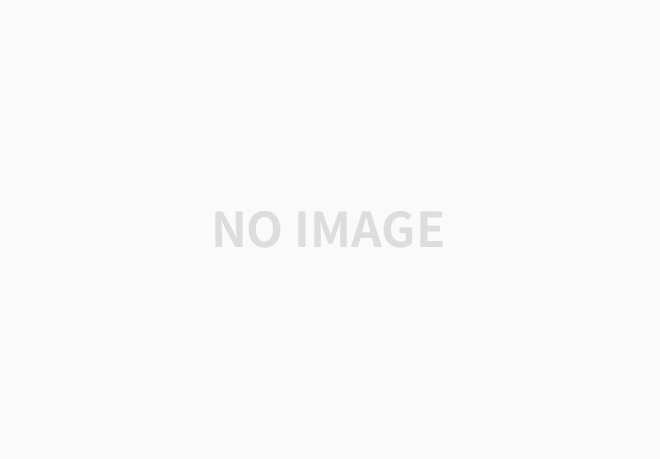
Parch와 SibSp는 딱히 패턴이 안보인다. 버리자.
1-5. 데이터 시각화
# 나이별 비생존자와 생존자
g = sns.FacetGrid(train_df, col='Survived')
g.map(plt.hist, 'Age', bins=20)
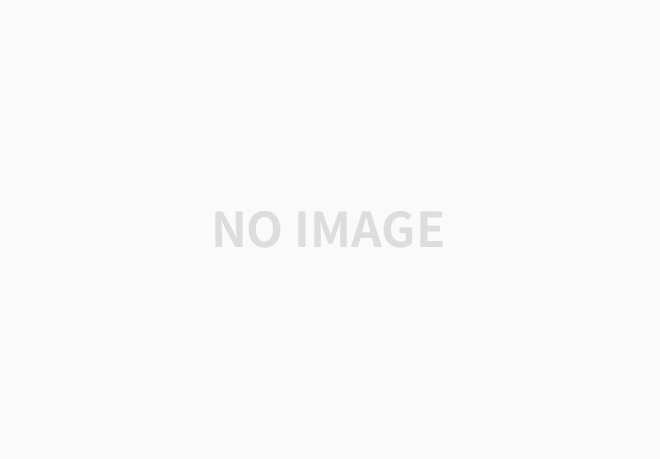
# 나이 및 객실 등급별 생존여부
grid = sns.FacetGrid(train_df, col='Survived', row='Pclass', size=2.2, aspect=1.6) # 행: 객실 등급, 열: 생존여부
grid.map(plt.hist, 'Age', alpha=.5, bins=20) // plt.hist, alpha = 투명도, bins = 갯수
grid.add_legend();
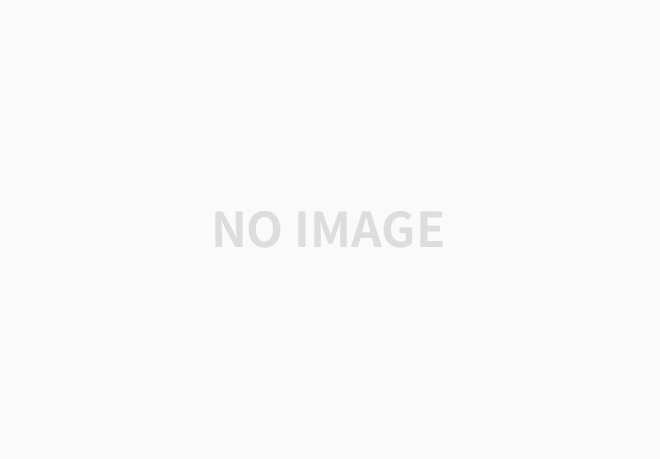
# 꺾은선 그래프
Embarked, PClass, Sex에 따른 생존 여부
grid = sns.FacetGrid(train_df, col='Embarked', size=2.2, aspect=1.6)
grid.map(sns.pointplot, 'Pclass', 'Survived', 'Sex', palette='deep', order=[1, 2, 3], hue_order=None)
grid.add_legend()
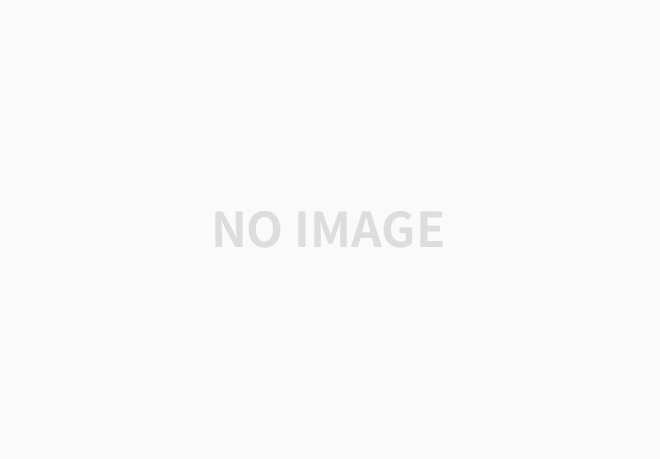
1-6. 데이터 전처리: 속성 조정
# 우선 현재 보유하고 있는 속성을 다시 한 번 확인해보자
train_df.head()
# 속성 조정
PassengerId는 샘플별로 다르기 때문에 제거
Survived: 예측해야할 output
Age, Fare: 그대로 채택
Sex, Pclass, Embarked: 카테고리 값이므로 처리.
SibSp, Parch: Binary 값으로 수정
Ticket: 표 번호이므로 상관성이 없는 값이라 제거
Name: 한 번 살펴볼 것.
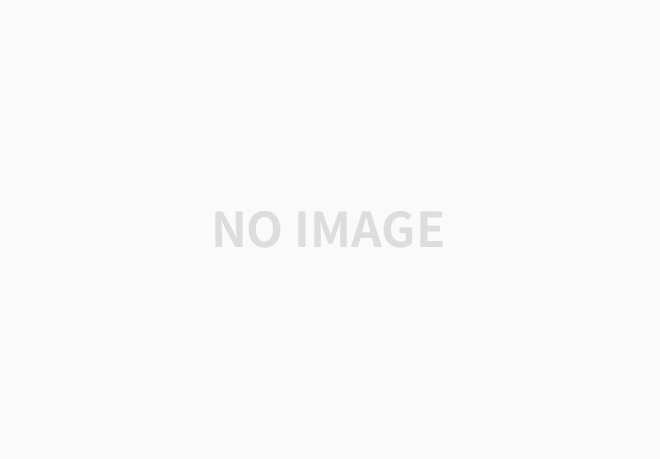
1-6-1. SibSp, Parch를 Binary 값으로 수정
# 신규 속성인 TravelSibSp, TravelParch 만들어줌
# 해당 속성이 0보다 크면 1, 아니면 0
train_df['TravelSibSp'] = np.where(train_df['SibSp']>0, 1, 0)
train_df['TravelParch'] = np.where(train_df['Parch']>0, 1, 0)
# 이후 SibSp, SibSp 제거
train_df.drop('SibSp', axis=1, inplace=True)
train_df.drop('Parch', axis=1, inplace=True)
# test 데이터도 마찬가지로 적용
test_df['TravelSibSp'] = np.where(test_df['SibSp']>0, 1, 0)
test_df['TravelParch'] = np.where(test_df['Parch']>0, 1, 0)
test_df.drop('SibSp', axis=1, inplace=True)
test_df.drop('Parch', axis=1, inplace=True)
1-6-2. Pclass, Embarked, Sex 처리
Pclass에 세 가지가 있으니 Pclass 라는 속성을 세 개로 쪼갠다. Pclass_1, Pclass_2, Pclass_3
Embarked도 마찬가지. S, C, Q 가 있으니 Embarked_S, Embarked_C, Embarked_Q
Sex도 마찬가지, female, male 이 있으니 Sex_female, Sex_femal
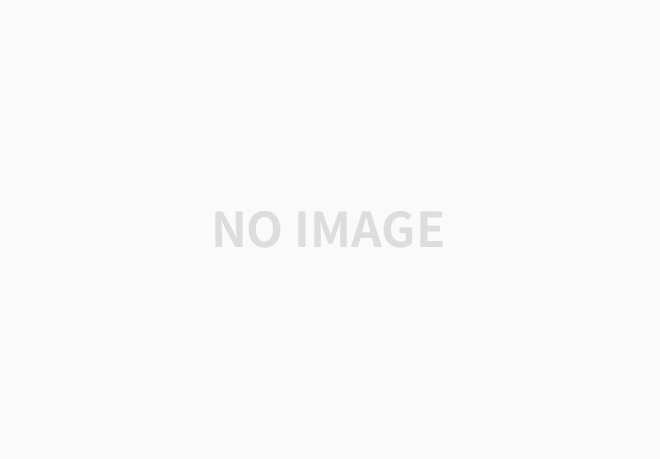
1-6-3. 쓸모없는 속성 제거
train_df4.drop('PassengerId', axis=1, inplace=True)
train_df4.drop('Name', axis=1, inplace=True)
train_df4.drop('Ticket', axis=1, inplace=True)
train_df4.drop('Sex_male', axis=1, inplace=True) // 어차피 성별은 0 아님 1이므로 하나 제거
train_df4.head() //
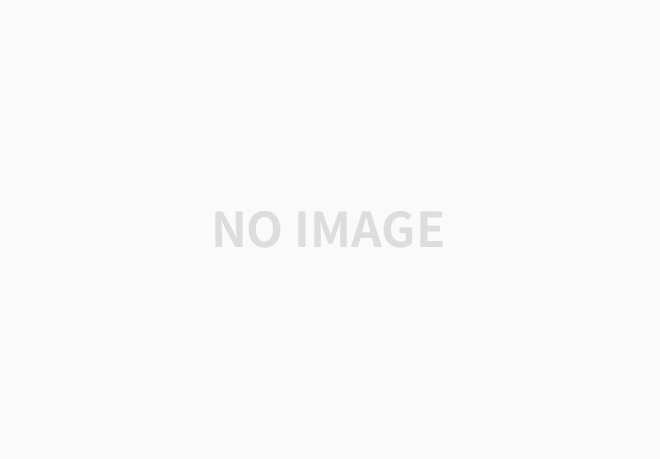
1-6-4. test_df에도 똑같이 해주자
test_df2 = pd.get_dummies(test_df, columns=["Pclass"])
test_df3 = pd.get_dummies(test_df2, columns=["Embarked"])
test_df4 = pd.get_dummies(test_df3, columns=["Sex"])
#test_df4.drop('PassengerId', axis=1, inplace=True) <--- 이건 나중에 평가를 위해 일단 지금은 지우지 말자
test_df4.drop('Name', axis=1, inplace=True)
test_df4.drop('Ticket', axis=1, inplace=True)
test_df4.drop('Sex_male', axis=1, inplace=True)
test_df4.head()
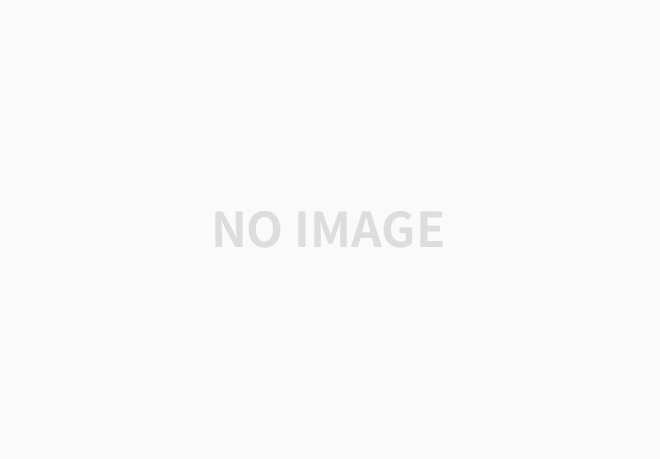
드디어 데이터 전처리 끝.
이제 ML(Machine Learning)을 이용하여 생존자를 예측해보자.
2. Machine Learning 활용한 생존자 예측
ㅇ 활용 모델
2.1 Logistic Regression
2.2 k-Nearest Neighbors
2.3 Support Vector Machines
2.4 Naive Bayes classifier
2.5 Decision Tree
2.6 Artificial neural network
2-0. 일단 학습집합과 테스트 집합 준비
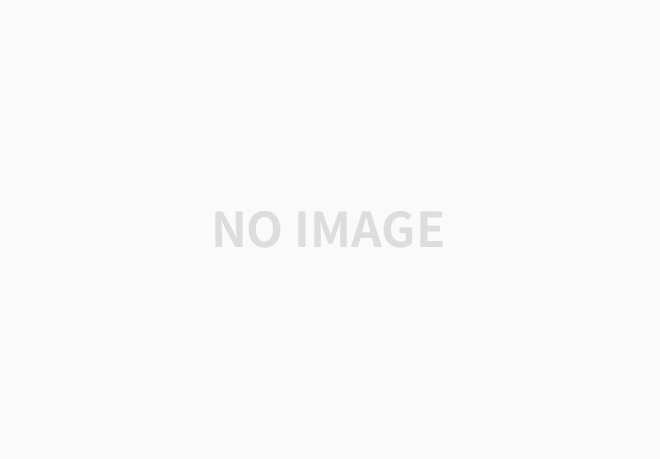
나머지는 졸려서 to be continued...
'직장생활 > Programming (C, Python)' 카테고리의 다른 글
[Python] Suicide Rates Overview 1985 to 2016 from Kaggle (0) | 2021.07.29 |
---|---|
[TensorFlow] 튜토리얼 - Keras basic - Basic classification: Classify images of clothing (0) | 2021.07.29 |
[데이터마이닝] Linear Regression_Python (0) | 2021.07.29 |
[Python] 엑셀(xlsx)파일 csv로 변환하기: python xlsx to csv (0) | 2021.07.29 |
[Python] 조건에 따른 dataframe 원소값 생성/변경/삭제 with loc(), str.contains() + np.where() (0) | 2021.07.29 |